Compact output from wttr.in
At this point I'm sure everyone is familiar with wttr.in, a neat little website that can give you a nice weather report in you terminal with:
curl http://wttr.in/uppsala
I wanted to use this in my daily automated morning email to myself but the output is too wide for my terminator i3wm scratchpad so I did an ugly Python hack to fix this.
From this:
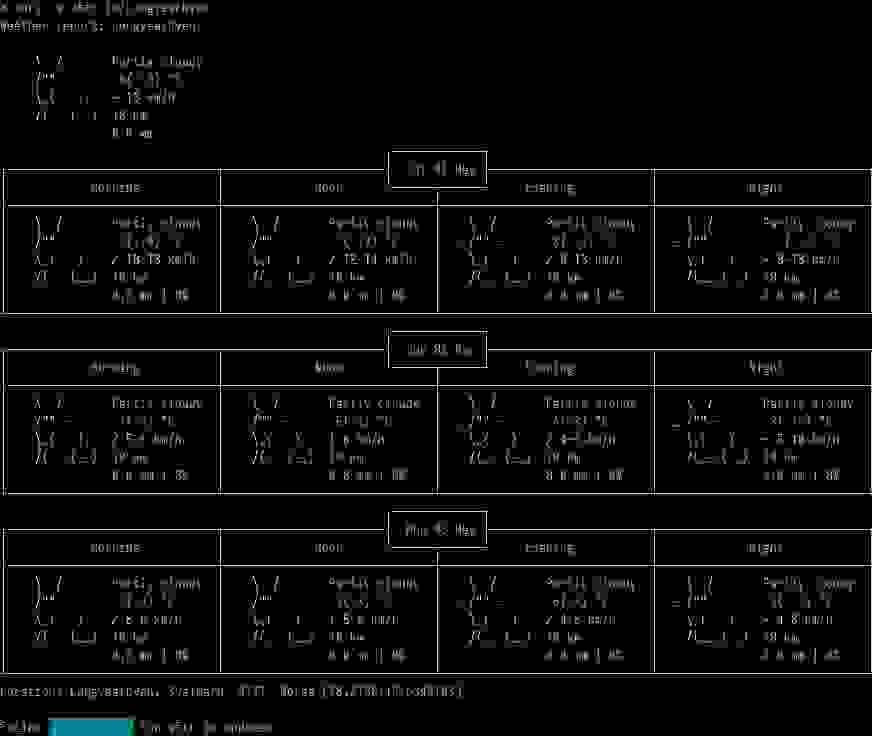
To this:
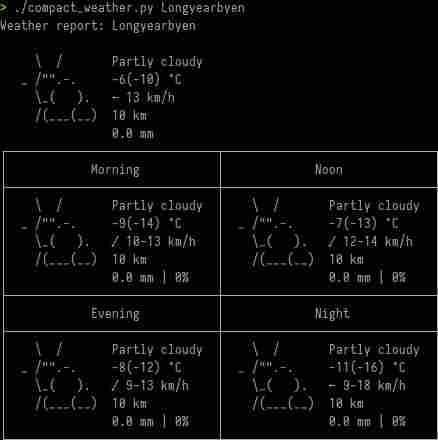
Much better for my purposes.
The code isn't very good and I am and probably always will be a Python n00b, but hey it works. In case anyone would find this useful I've created a repository on github: compactwttr.
In fact the code is concise enough to fit in this post.
#!/usr/bin/env python3 """ A small script get weather infom from wttr.in via curl and remove colors and compact the output. """ import subprocess import re import sys def split(tail): """ splits the tail wrt the boxes """ chunk1 = [] chunk2 = [] for line in tail: chunk1.append(line[:63]+'\n') chunk2.append(line[63:]) for i in chunk2[1:]: i = "│"+i+'\n' chunk1.append(i) return chunk1 def fix_formatting(chunk): """ fixes the quirks in the formatting """ chunk[0] = chunk[0][:55]+"───────┐\n" chunk[1] = chunk[1][:55]+" │\n" chunk[2] = chunk[2][:-2]+"┤\n" chunk[8] = "├"+chunk[8][1:31]+"┼"+chunk[8][32:-2]+"┤\n" chunk[9] = chunk[9][0]+" "+chunk[9][8:] chunk[10] = "├"+chunk[10][1:] chunk[16] = "└"+chunk[16][1:] return chunk def remove_colors(string): """removes ANSI color codes from string https://stackoverflow.com/a/14693789/6586742 """ ansi_escape = re.compile(r'\x1B(?:[@-Z\\-_]|\[[0-?]*[ -/]*[@-~])') result = ansi_escape.sub('', string).split('\n') return result def main(): """ all args to string. start curl subprocess to get data, remove colors, compact and fix output""" city = ' '.join(sys.argv[1:]) cmd = ["curl", "-s", "wttr.in/"+city] output = subprocess.check_output(cmd).decode('utf-8') output = remove_colors(output) head = "\n".join(output[:7]) tail = output[8:-24] tail = split(tail) tail = fix_formatting(tail) tail = "".join(tail) print(head+'\n'+tail) if __name__ == "__main__": main()